Automated testing – is an integral part of modern software development that helps Agile teams make software reliable and well-performed.
However, managing and maintaining test scripts can be a daunting task, especially when working on large-scale projects. That’s why opting for the Page Object Model (POM) popular design pattern is necessary. Page Object Model not only offers a structured approach to automated testing but also simplifies script maintenance and management.
With POM in place, QA Automation teams can better structure and optimize large test suites. They can reuse its code with methods and variables for updating and modifying the application’s user interface without making extensive changes to the test logic. This contributes to more fast, robust and scalable processes in the authoring and maintenance of automated testing framework. Let’s deep into more detail 👀
What exactly is Page Object Model Pattern?
Being a broadly embraced design pattern in automated testing, the Page Object Model (or POM) represents a part of the web application. An e-commerce web application might have a home page, a listings page, a checkout page and a contact page. Each of them can be represented by page object models.
Page objects are a classic example of encapsulation – they hide the details with methods and variables to not clutter the tests.
At the core of Page Object Models pattern lies the concept of creating a class file for each webpage or component within a web app. Each class file accommodates only corresponding web page elements. These classes encapsulate the user interface (UI) elements and their functionalities. Each page object represents a page within the web application and includes methods to interact with the web elements presented on that specific web page, like buttons, checkboxes or text fields (for example, password field).
For instance, an E-shop page showing multiple product cards would have a section list page object containing several cards. There would probably also be a header page object and a footer page object.
Similarly, if we navigate to another page, the initial page object should return another page object for the new page. In general page object operations should return fundamental types (strings, dates) or other page objects.
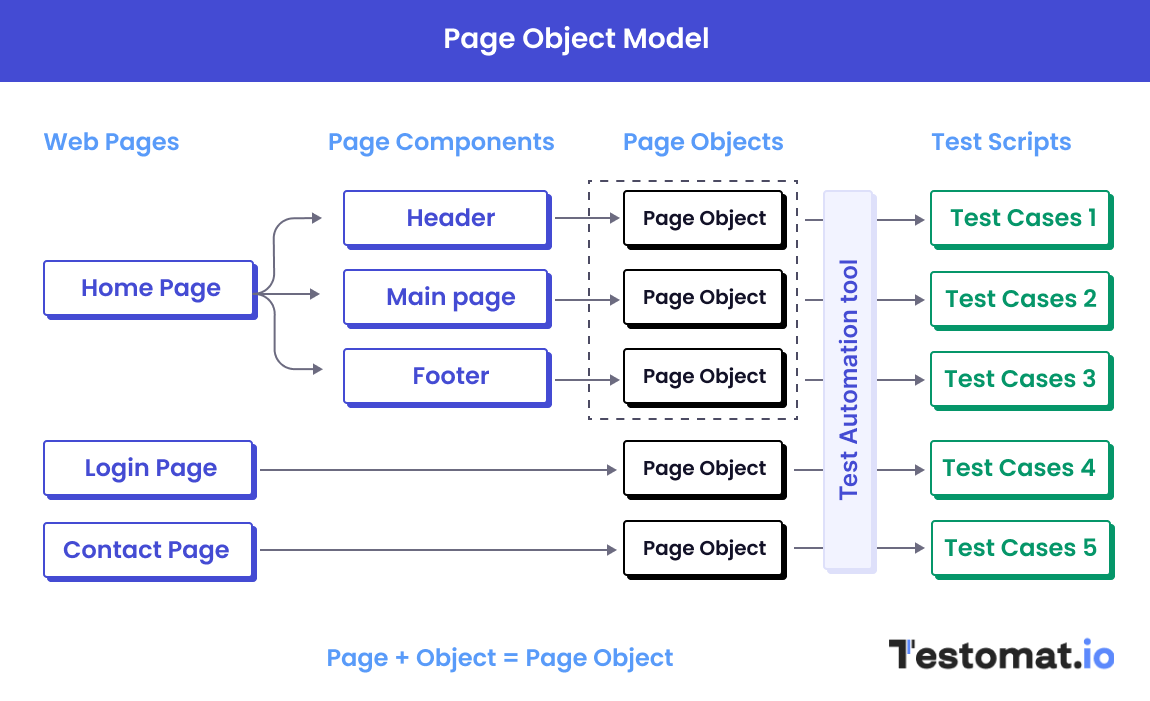
Despite the term Page Object, these objects should not usually be built for each page, but rather for the significant elements on a page. The rule of thumb is to model the structure in the page that makes sense to improve our testing.
Thus modelling the application’s user interface (UI) into a dedicated object called a Page Object helps teams not only get code duplication low but also improve the test framework overall.
What are the key components of the Page Object Model?
Here you can find the key components and concepts for Page Object Model (POM) pattern implementation offered by the modern test automation solutions that will help you better understand the process:
Page Object’s Classes
As the core components of the POM pattern, they are represented by a class and correspond to each page of the application. These objects encapsulate the behavior and structure of the webpage.
UI Element Locators
These web elements represent the various components on a webpage, including buttons, input fields, checkboxes, etc. Also, they contain references to other UI elements and links.
Locators are used to find these web elements in equivalent. Locators include CSS selectors, XPath expressions, IDs, class names, etc. This helps webpage objects uniquely define and interact with specific UI elements.
Test Methods
With test method, you can simulate user interactions with the application by utilizing the webpage objects and their associated actions. By utilizing methods from UI objects, tests can be written clearly and concisely to focus on the test scenario logic.
Separation of Concerns
This practice divides the codebase into manageable and coherent sections to address a separate concern or responsibility. Objects on the page encapsulate the UI interactions, while test scripts focus on test logic and flow. This separation provides independent testing, development and modification of different sections without affecting other parts of the application.
Action Methods
Objects contain methods that represent actions such as clicking a button, filling a form, or navigating to another web page. These methods make the automation scripts more readable and maintainable.
Parameterization
With parameterization, you can customize or provide specific details when using actions in your test cases without duplicating code.
Assertions
Webpage objects may include assertion methods to validate the expected behavior of the application and verify that it functions as intended.
Navigation Methods
Page objects can have methods for navigating within the web application, facilitating seamless transitions between different pages or components.
What is Playwright?
Playwright is a powerful and robust automation framework that simplifies browser automation. Built by Microsoft, Playwright supports various programming languages, including JavaScript. Applied to simplify browser automation and increase the quality of web application testing, this tool allows teams to execute tests in both headless and headful modes.
With given access to the Debugger mode, software engineers can leverage this capability to deeply analyze and troubleshoot issues and anomalies to ensure the app is error-free. What’s more, coupled with a host of other features, Playwright is considered to be a go-to choice for modern browser automation.
Learn more with posts:
- Playwright vs Cypress: Which Platform to Choose for Web App Testing
- 🎭 Test Automation with Playwright: Definition and Benefits of this Testing Framework
- How To Capture Screenshots & Videos — Playwright JS Tutorial
- Playwright Testing Tutorial on How to Organize an Advanced (Scaled) (e2e/unit) Testing Framework
Why do teams choose Playwright technology along with POM?
Let’s find out why this tool is a good option to incorporate POM (Page Object Models pattern):
- Teams can write tests and make sure the app works consistently across different browsers.
- With a unified API provided by Playwright, you can write one set of UI objects and scripts that remain consistent and seamlessly work across all supported browsers.
- With a rich set of features, including interactions with each web element, file downloads, handling dialogs, etc., teams can implement the desired app behavior more easily.
- Thanks to Playwright’s high performance and reliability, teams can execute tests quickly and with stable interactions.
- Playwright’s built-in support for async operations aligns well with POM implementation.
- Teams learn and integrate POM effectively thanks to documentation, tutorials, and a supportive community.
- Teams can implement custom utility functions to manage and organize web element interactions.
How to Implement the POM Pattern with Playwright
To effectively incorporate the Page Object Model with Playwright tool, you should set up your project. Typically, you need to organize UI objects for each webpage or component within the web application. They encapsulate the page’s interactions and functionalities, providing reusable methods to automate tests. Here’s a step-by-step guide to help you implement the sample project structure for POM with Playwright and perform actions effectively:
# 1 Step: Set Up Your Project
At the very start, create a new folder for your project and initialize a new Node.js project:
npm init
Then you need to install and setup Playwright as a dependency in your project by writing:
npm init playwright@latest
#2 Step: Record our first test
Let’s create our first test, we can use Playwright Test Generator for this purpose:
npx playwright codegen
Also, we use Demo access to sign in to Orange CRM and create our first Playwright test like in screen:
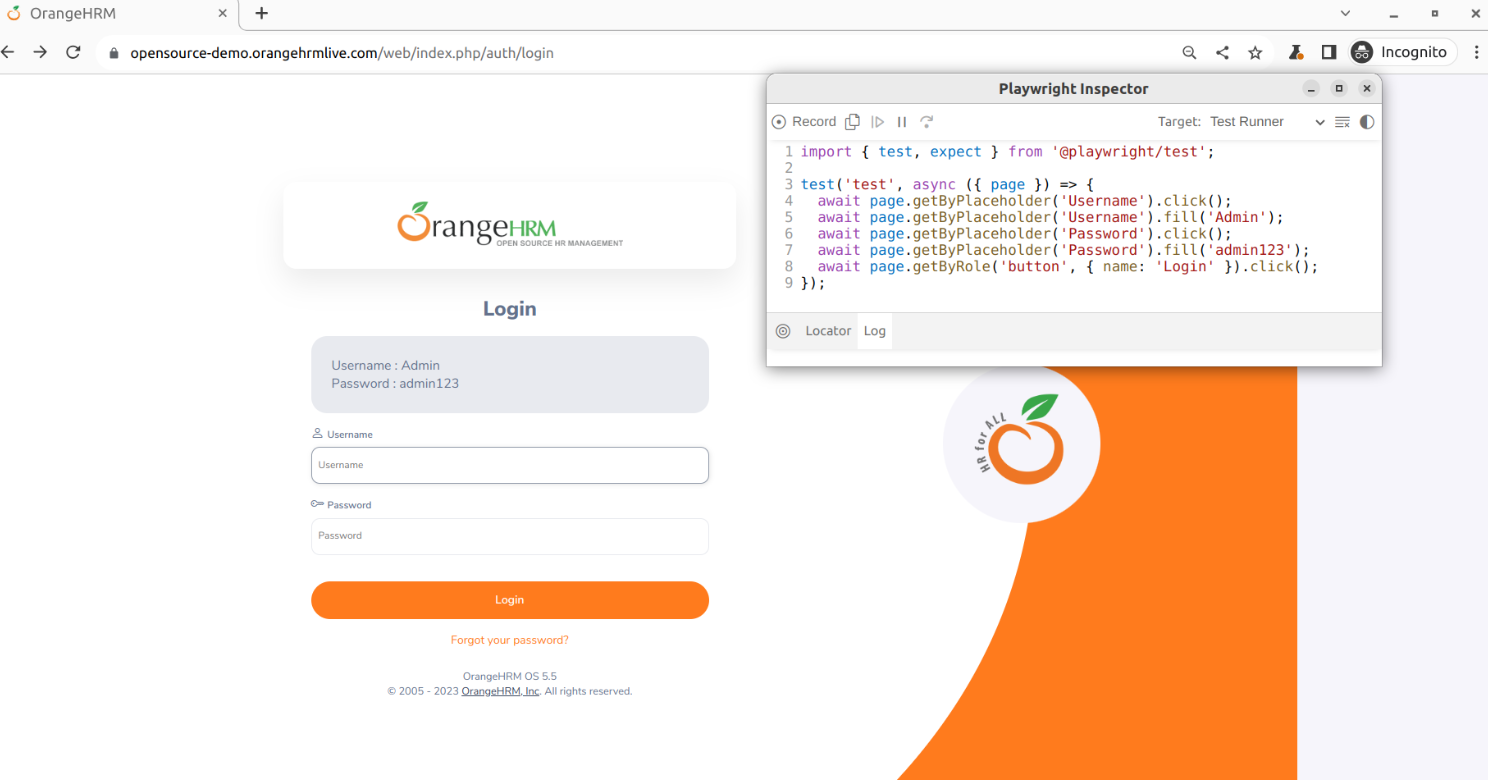
#3 Step: Create Web Page Object or New Web Element
For each web page or component, you should create a separate JavaScript object class that represents a page object. These classes will encapsulate the interactions with the respective web pages.
exports.LoginPage = class LoginPage {
constructor(page) {
this.page = page
this.username_field = page.getByPlaceholder('Username')
this.password_field = page.getByPlaceholder('Password')
this.login_button = page.getByRole('button', { name: 'Login' })
}
async gotoLoginPage() {
await this.page.goto('https://opensource-demo.orangehrmlive.com/web/index.php/auth/login');
}
async login(username, password){
await this.username_field.fill(username);
await this.password_field.fill(password);
await this.login_button.click()
}
}
We have created a new folder pages and file login.js where we wrote these classes. Check out the initial Demo Playwright project structure now:
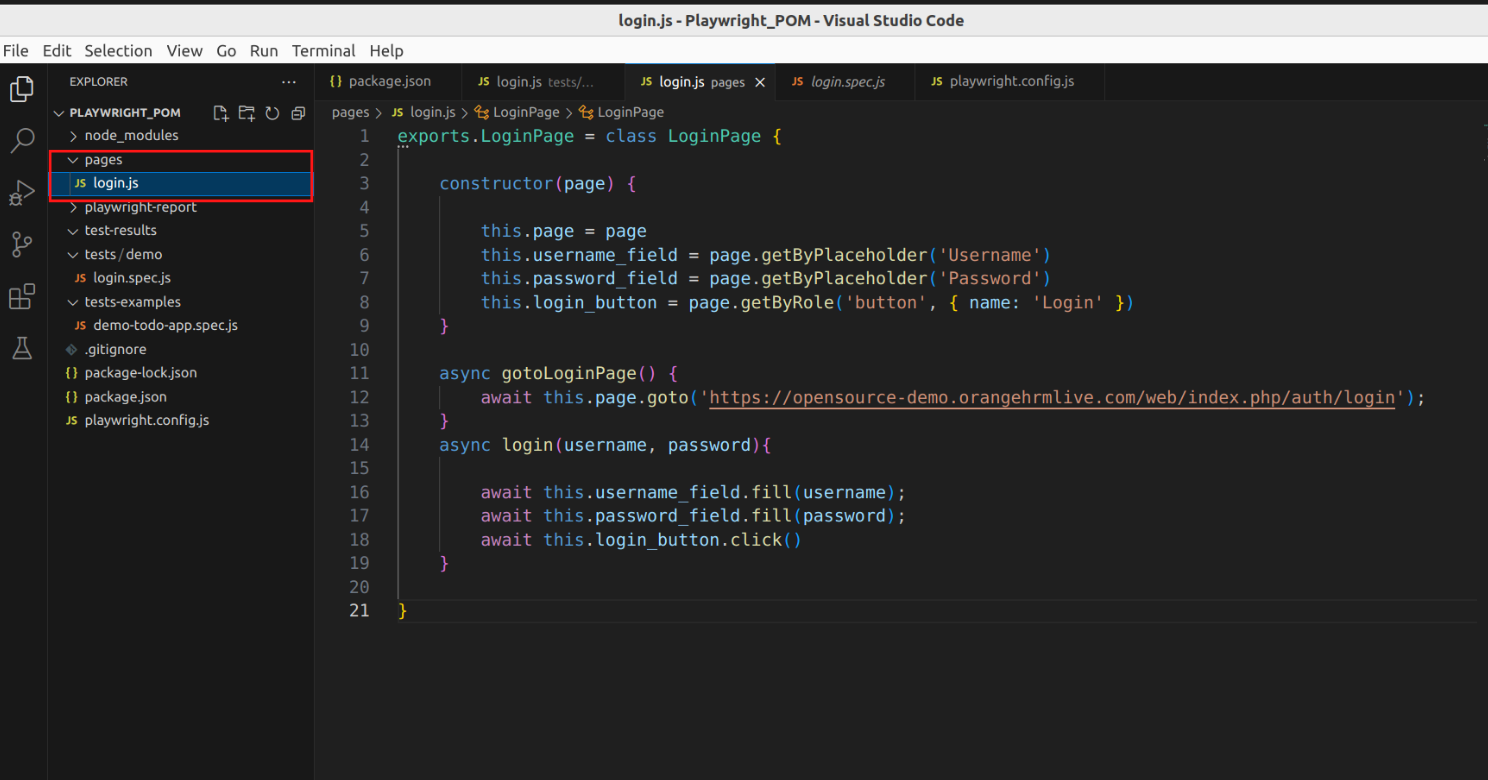
#4 Step: Use Page Objects in Test Scripts
At this step, you need to write test automation scripts that utilize the webpage objects to interact with the application. You need to remember that these scripts should be concise and easy to read. This will improve the overall maintainability of the automation code. So, we rewrite our first test as follows below:
import { test } from '@playwright/test';
import {LoginPage} from '../../pages/login.js';
test('test', async ({ page }) => {
const Login = new LoginPage(page)
await Login.gotoLoginPage()
await Login.login('Admin', 'admin123' )
});
#5 Step: Execute the Test
Execute the test using Playwright’s test runner to observe the automated interactions with the application.
npx playwright test
By default, Playwright test runs in headless mode. You should use a headed flag to run in headed mode.
#6 Step: Maintain and Expand
As the application grows, you should maintain and update the page object methods to reflect any changes in the UI. In addition to that, it is crucial to create new webpage objects or extend existing ones to support new features or pages.
We hope that the presented steps will help you implement the Page Object Model with Playwright and JavaScript to drive efficient and maintainable automated testing for your application.
What are the benefits of the POM Pattern?
For any organization striving to enhance the testing process, turning to the Page Object Pattern can foster automated testing and provide better results. There are numerous benefits of the Page Object Models pattern that will help organizations meet with success. Let’s take a closer look and understand the value it provides:
- With pre-built objects on the web page and standardized methods for interacting with the UI web element, POM speeds up test script development. QA engineers can utilize these objects without the need to create UI interaction functions from scratch making the process faster and more efficient.
- POM model helps teams keep their code clean, readable, and well-organized.
- POM provides easy maintenance and eliminates the need to use the same web element at several tests, by centralizing all UI-related actions and locators in webpage objects,
- Separating UI interactions from test logic provides test scripts readability with a focus on the test flow and scenario logic.
- POM improves collaboration among developers and testers. While testers are creating or modifying test automation scripts using objects on the page, developers are working on the page object classes. At the same time, front-end developers can update locators in the UI objects.
- Thanks to POM, any changes or updates made in a centralized location minimize redundancy and provide consistency.
- POM allows teams to isolate the problem (if found) to a specific page object and debug it. This significantly reduces the time taken to identify and fix issues.
- With well-designed UI objects, teams can incorporate error-handling mechanisms to manage exceptions and unexpected behaviors of the app as well as ensure robustness in the test suite.
However, you should take into account that your team should have good coding skills to set the POM framework. And you need to remember that the initial design takes some time to be implemented.
What Are The Challenges Of The Page Object Model?
While POM offers many benefits, it also comes with its set of challenges. So, it’s crucial to be aware of potential difficulties that might arise during its implementation:
- Initial setup complexity: When dealing with complex web applications, you may have nested page objects or components as Page Object Hierarchies. Managing the hierarchy of page objects and their relationships can be challenging and may require careful design. So arranging is necessary to ensure effective page element mapping and structuring.
- Abstraction Level: Striking the right balance in terms of the abstraction level of page objects can be challenging. If they are too high-level, they may not provide enough flexibility for various test scenarios, while if they are too low-level, they can lead to code duplication and maintenance problems.
- Page Object Naming: Choosing meaningful and consistent names for page objects is crucial. Inconsistencies in naming can make it difficult to locate and use page objects, especially in larger test suites.
- Synchronization Issues: Test automation scripts need to interact with web elements that might not be immediately available or fully loaded when the script attempts to access them. Implementing proper synchronization mechanisms in the page objects to handle these timing issues can be challenging. Especially it is true when dealing with dynamic web elements.
- Cross-Browser and Platform Compatibility: It can be challenging to guarantee that page objects and tests function correctly across different browsers and platforms. Browser-specific issues and platform variations need to be addressed in your page objects.
- Parallel Execution: Implementing parallel test execution can be challenging, as you need to ensure that page objects and tests do not interfere with each other when running concurrently.
- Maintenance: POM requires continuous maintenance as the web application evolves. When elements on a web page change, you need to update the corresponding page objects, which can be a tedious and ongoing task.
- Adapting to changes: POM makes managing modifications simpler. Nevertheless, it can still take time to get used to frequent UI changes or large application updates.
- Consistency across teams: Ensuring that POM practices are applied consistently across various teams and projects might be difficult. It may take some time for team members to become proficient in using page objects efficiently.
Despite these challenges, the Page Object Model remains a valuable design pattern for test automation.
Best Practices for Effective POM Implementation
When it comes to the POM pattern implementation with Playwright, it is crucial to consider the following best practices:
- Choosing meaningful and easy-to-understand names for methods and UI objects on the page is essential to enhance the readability and maintainability of your Page Object Model structure.
- Applying reliable and unique locators to identify and initialize web elements will help you generate stable and robust test automation scripts.
- Do not forget to implement error-handling mechanisms within UI objects in order to effectively manage unexpected situations.
- It would be best if you kept business logic out of objects located on the page to easily manage and maintain the app when the UI-related operations are distinct.
- You need to create reusable utility methods to handle common actions and speed up the testing process.
Bottom Line: Want to foster automated testing with the POM Pattern?
Implementing the Page Object Model (POM) pattern with Playwright and JavaScript opens up ample opportunities for automated testing. It not only drives a well-organized and scalable testing framework but offers a structured approach to automated testing.
In addition to that, POM improves code readability, reusability, and collaboration among team members by encapsulating UI interactions into reusable page objects.
Moreover, Playwright’s unified API enhances POM by simplifying browser interactions and providing consistent and rigorous testing across different browsers. With that in mind, the combination of POM and Playwright empowers teams to streamline their testing efforts and contributes to more effective and reliable testing processes in your workflow.
As technology continues to evolve, the Page Object Model framework is poised to undergo exciting advancements that can further streamline automated testing processes:
The future trend of the Page Object Model pattern is the development of page items can be automated with AI. Artificial Intelligence algorithm recommendations allow AQAs to save time and effort. Furthermore, it can also aid in analyzing user interface changes and producing related page items.
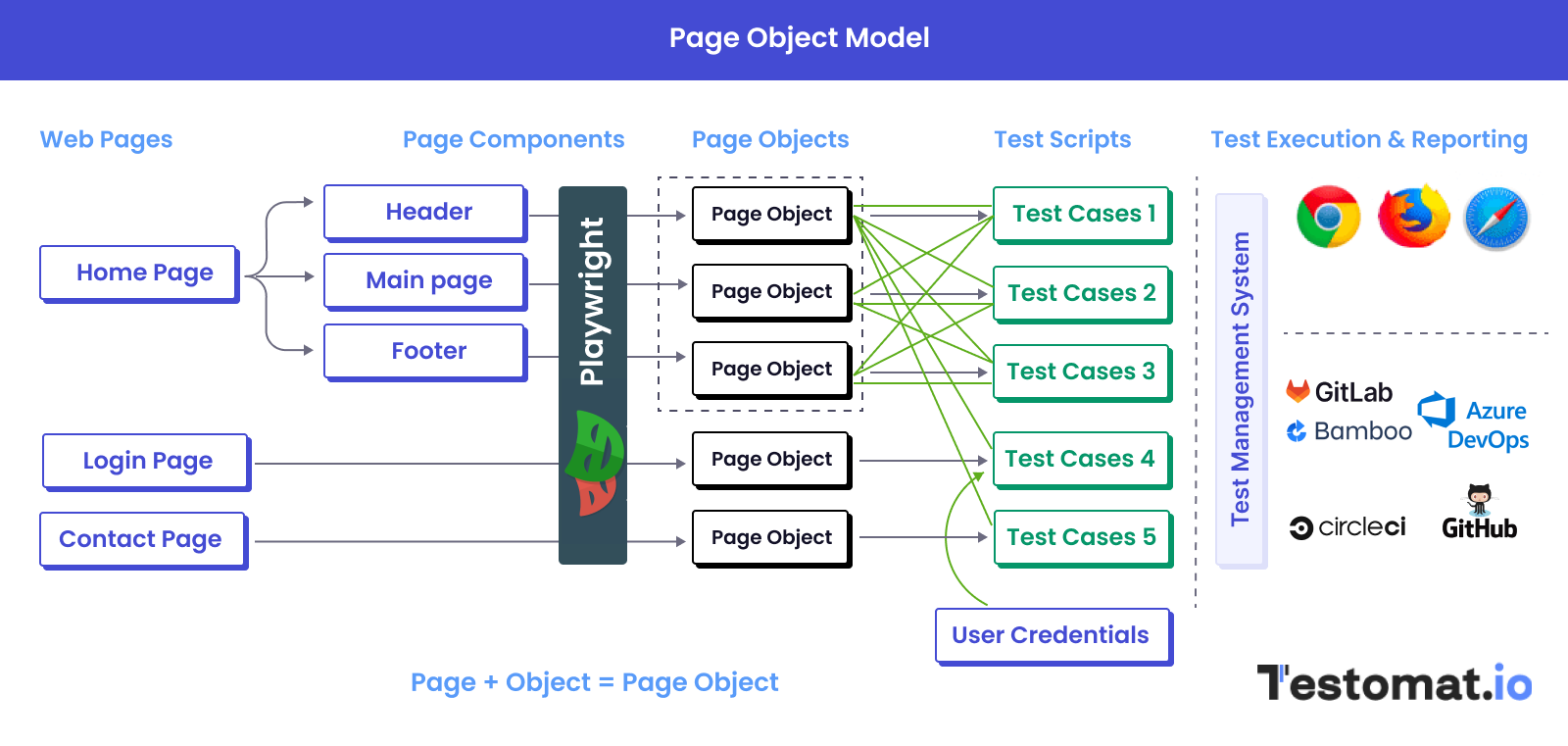
💡 If you have any questions, feel free to ask us. We are ready to help you integrate your Playwright tests and make automation testing easier through robust design patterns. Our advanced Reporting and Analytics tools with CI\CD could provide insights into the effectiveness of POM-based tests. Furthermore, analytics could suggest areas for optimization and test automation coverage improvements.